Introduction
In the realm of C programming, efficient memory management is paramount. Unlike some languages with automatic garbage collection, C++ provides developers with the power (and responsibility) of dynamic memory allocation. This means programmers can allocate blocks of memory during program execution as needed, leading to optimised memory usage. However, this flexibility comes with the risk of memory-related errors if not handled properly.
Key Highlights
- Efficient memory management is critical for C++ programs to avoid issues like memory leaks and dangling pointers.
- Dynamic memory allocation allows flexibility in managing memory, allocating it during runtime as needed.
- The
new
anddelete
operators are fundamental for dynamic memory allocation and deallocation in C++. - Smart pointers, such as
unique_ptr
andshared_ptr
, automate memory management and help prevent common errors. - Understanding memory leaks, dangling pointers, and double deletion is essential for writing robust C++ code.
Understanding Memory Management in C++

Memory management is the process of controlling how a program accesses and uses computer memory. In C++, this involves allocating memory from the operating system for data storage and releasing it back to the system when it is no longer needed. Proper memory management is crucial for several reasons.
Firstly, it ensures that a program only uses the memory it needs, which is particularly important in resource-constrained environments. Secondly, it helps prevent memory leaks, a situation where a program continuously consumes memory without releasing it, eventually leading to performance degradation or even system crashes.
Importance of Efficient Memory Management
Efficient memory management is critical for C++ programs to function correctly and efficiently. During program execution, the operating system allocates a certain amount of main memory to the program. This memory is used to store the program’s code, static data, and dynamic data. Without efficient management, programs can encounter serious issues.
One primary concern is the potential for “memory leaks.” When a program fails to release allocated memory that is no longer in use, it ties up resources that could be used by other parts of the program or the operating system itself. Over time, this can lead to a shortage of available memory, causing performance degradation or even crashes.
Moreover, inefficient memory management can also lead to security vulnerabilities. By not properly releasing sensitive data from memory, malicious actors could potentially exploit these remnants, compromising the integrity of the application and user data.
Key Concepts in C++ Memory Management
To effectively manage memory in C++, one must be familiar with key concepts. The memory management unit (MMU) of the operating system plays a crucial role in allocating and deallocating blocks of memory to a program.
When a program needs memory, it can dynamically request a block of a specific size during runtime. Once allocated, the program can access and modify the data within this memory block using pointers. However, it’s the program’s responsibility to track this allocated memory.
Failure to release unused, allocated memory leads to memory leaks, potentially causing performance issues or crashes over time. On the other hand, attempting to access memory that has already been deallocated leads to undefined behavior, often resulting in program crashes or unpredictable results.
Dynamic Memory Allocation in C++
At the heart of C++ memory management is dynamic memory allocation. This mechanism empowers programs to request and release memory during runtime, rather than having a fixed amount determined at compile time. This flexibility is vital for handling data structures and scenarios where the memory requirements change dynamically based on user input or program logic.

C++ provides powerful tools for dynamic memory allocation: the new
and delete
operators. These operators provide greater control and efficiency compared to older C-style memory allocation functions like malloc
and free
.
The Role of new and delete Operators
New and delete operators in C++ play a crucial role in dynamic memory allocation. The new operator is used to allocate memory during runtime, while delete is utilized to release that memory. When a C++ program requires a block of memory, these operators come into play, ensuring efficient use of allocated memory. Understanding the nuances of new and delete operators is essential for effective memory management in C++ programs. By mastering their use, developers can optimize memory usage and prevent memory leaks.
Smart Pointers and Their Impact on Memory Management
Smart pointers are a powerful feature in C++ that simplifies memory management and helps prevent errors. Unlike raw pointers, which only store memory addresses, smart pointers wrap these raw pointers within a class, providing automatic memory management.
Acting as a layer of abstraction over raw pointers, smart pointers help avoid common pitfalls like memory leaks. They do this by tying the lifetime of the allocated memory to the scope of the smart pointer object itself. Let’s look at some key benefits:
- Automatic deallocation: Smart pointers automatically deallocate the memory they manage when it’s no longer needed, preventing memory leaks.
- Exception safety: In case of exceptions during program execution, smart pointers ensure proper memory deallocation, preventing resource leaks.
- Improved code readability: Smart pointers make code cleaner and easier to understand by abstracting away manual memory management details.
Common Pitfalls and How to Avoid Them
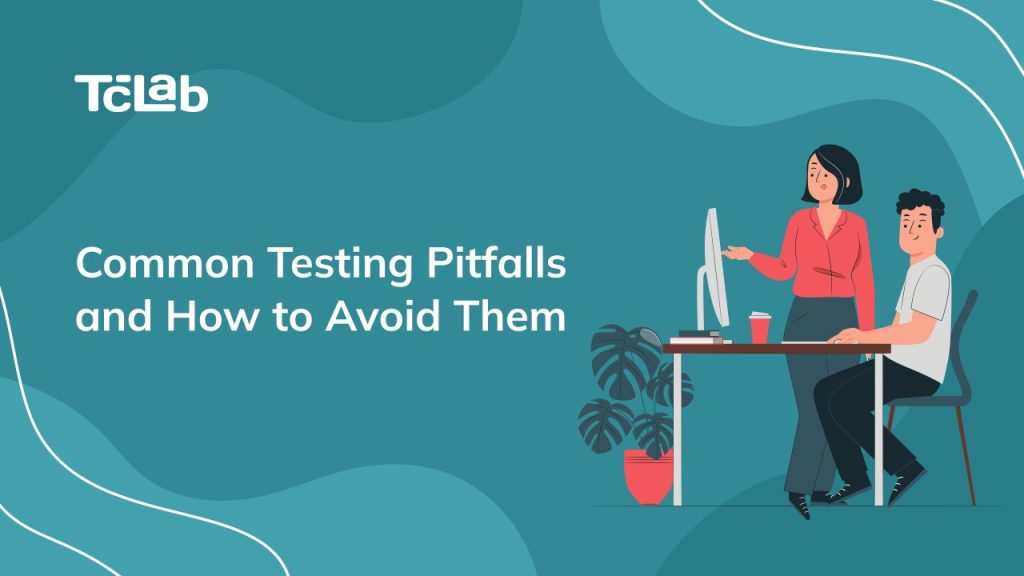
Dynamic memory allocation, while powerful, is not without its pitfalls. Programmers must tread carefully to avoid introducing memory-related errors into their C++ code. Failure to properly manage allocated memory can lead to a range of issues, from subtle performance degradation to catastrophic program crashes.
This section will cover some of the most common memory management pitfalls and provide clear strategies for avoiding them. Understanding these potential issues is crucial for writing robust and reliable C++ applications.
Memory Leaks and Their Detection
A memory leak is like a slow drip that can eventually flood a system. It occurs when a program allocates memory but fails to release it back to the operating system when it’s no longer needed. Over time, these unreleased blocks of memory accumulate, reducing the amount of available memory for other processes.
Detecting memory leaks can be tricky as they often don’t manifest immediately. In the beginning, the impact may be negligible. However, as the program runs for extended periods or handles large datasets, the leaked memory starts to add up, eventually leading to performance degradation, instability, or even system crashes.
There are several tools and techniques for detecting memory leaks in C++ applications. Memory debuggers, for example, can track memory allocations and deallocations, identifying blocks of memory that have been allocated but not deallocated. Additionally, static analysis tools can analyze source code to identify potential memory leak patterns.
Dangling Pointers and Double Deletion
Dangling pointers and double deletion are two more common pitfalls in C++ memory management that can lead to program crashes and unpredictable behavior.
A dangling pointer occurs when a pointer points to a memory location that has already been freed. This can happen when the memory block is deallocated, but the pointer is not updated to reflect this change, resulting in a pointer pointing to an invalid memory location. Accessing memory through a dangling pointer can lead to unpredictable results.
Double deletion, on the other hand, happens when delete
is called twice on the same memory location. This attempt to free memory that has already been freed leads to corruption of the heap memory structure, causing undefined behavior, crashes, or unpredictable results in the program.
To avoid these errors, it’s crucial to ensure that pointers are updated after freeing the memory they point to, and to carefully manage the lifetime of allocated memory to prevent double deletion.
Conclusion
In mastering memory management in C++ programs, efficient memory handling is crucial for program performance and stability. Understanding key concepts like dynamic memory allocation, new/delete operators, and smart pointers is essential to avoid common pitfalls such as memory leaks and dangling pointers. By implementing proper memory management practices, you can enhance your program’s reliability and efficiency. Remember, staying vigilant about memory usage and applying best practices will lead to more robust C++ programs. If you want to delve deeper into optimizing memory management in your C++ projects, explore our FAQs or seek expert guidance for tailored solutions.
Frequently Asked Questions
What is memory management and why is it important?
Memory management is the process of allocating, using, and releasing memory in a computer program. Efficient memory management, involving the careful allocation and deallocation of memory for various data types, is crucial for optimal program performance and for preventing errors that can crash the entire program or even the operating system.
How do smart pointers facilitate memory management?
Smart pointers simplify memory management in C++. They act as wrappers around raw pointers, providing automatic deallocation of allocated memory when it is no longer in use. This automation relieves developers from the burden of manual memory management, reducing the likelihood of memory leaks.
Can memory leaks be completely avoided in C++?
While minimizing memory leaks is achievable through diligent programming practices and tools like smart pointers, completely avoiding them in dynamic memory allocation can be challenging. They occur when memory allocated on the heap using the new operator is not properly deallocated using delete, and even experienced programmers can make mistakes.